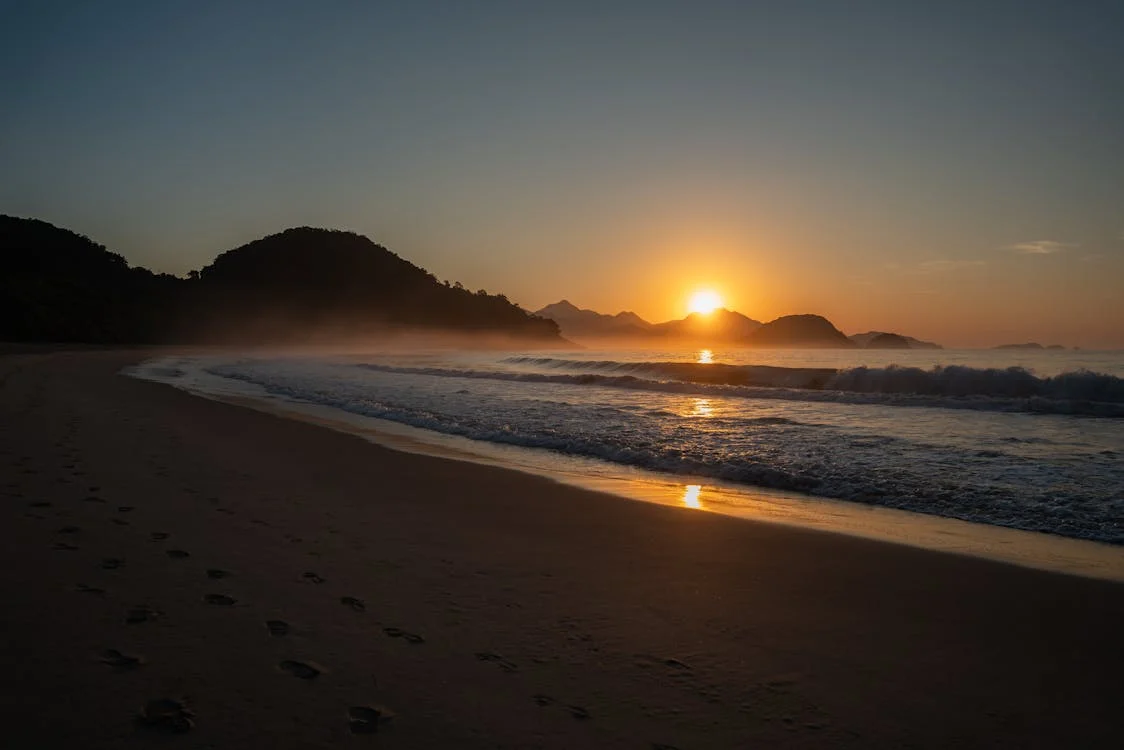
ZoomAny.js is a JavaScript library for zooming any HTML Element, including Images, UI Elements and Videos.
Get Started
Install
Install via NPM:
npm install zoom-any-js
Or via CDN:
CSS: https://cdn.jsdelivr.net/npm/zoom-any-js@latest/dist/zoom-any-js.css
JS: https://cdn.jsdelivr.net/npm/zoom-any-js@latest/dist/zoom-any-js.js
Basic Usage
To get started, add the class "zoomable" to the element you want to enable zoom on:
<img class="zoomable" src="public/img.png">
NPM + Bundler:
import ZoomAnyJs from "zoom-any-js";
import "zoom-any-js/dist/zoom-any-js.css";
const zoom = new ZoomAnyJs()
CDN:
<link type="text/css" rel="stylesheet" href="https://cdn.jsdelivr.net/npm/zoom-any-js@latest/dist/zoom-any-js.css">
<script type="module">
import ZoomAnyJs from "https://cdn.jsdelivr.net/npm/zoom-any-js@latest/dist/zoom-any-js.js"
const zoom = new ZoomAnyJs()
</script>
Now try to zoom into your image, it should start to zoom!
Docs
Methods:
Method | Description | Parameters | Returns |
---|---|---|---|
constructor | Selects the DOM element to be manipulated using the provided selector. Adds event listeners and applies zoom. | string (default=".zoomable") | void |
reset | Resets the internal values of the zoom element to their default state. | None | void |
getZoom | Retrieves the current zoom level of the element. | None | number |
setZoom | Sets the current zoom level of the element. | number | void |
setPos | Sets the position values (x and y coordinates) for the element. | object {x: number, y: number} | void |
getPos | Retrieves the current position values (x and y coordinates) of the element. | None | object {x: number, y: number} |
center | Centers the element within its container, which can be the window or the element's parent. | None | void |
fitToBounds | Adjusts the position of the element to fit within its bounds based on the data-origin and data-bounds attributes. | None | void |
zoomAt | Zooms the element based on the given amplitude and position. | number, object {x: number, y: number} | void |
addListeners | Adds event listeners to the element for handling user interactions. | None | void |
removeListeners | Removes event listeners from the element to stop handling user interactions. | None | void |
apply | Applies the current transformation and position values to the element. | None | void |
destroy | Removes all listeners and CSS changes, reverting the element to its initial state. | None | void |
Data-Options:
Attribute | Function | Default |
---|---|---|
data-max-zoom | Set maximum zoom | 4000 |
data-min-zoom | Set minimum zoom | 10 |
data-bounds | Enable fit to bound | false |
data-origin-parent | Use the offsetParent as origin for fitting bounding. Else it uses window | false |
Examples
Fullscreen
See the Pen ZoomAny.js Fullscreen by Ben Herbst (@BenHerbst) on CodePen.
Standalone
See the Pen ZoomAny.js Fullscreen by Ben Herbst (@BenHerbst) on CodePen.
Wrapper
See the Pen ZoomAny.js Standalone by Ben Herbst (@BenHerbst) on CodePen.